gulp.js で webpack 4 を呼び出す
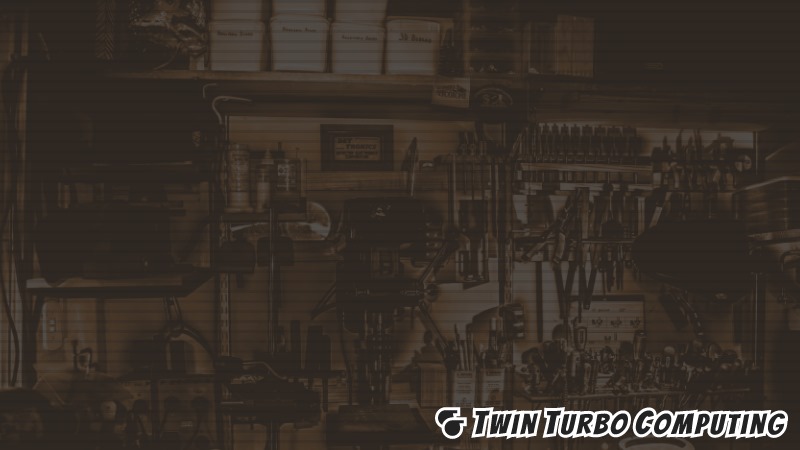
はじめに
今日は gulp を使って webpack を実行する方法をご紹介します。
webpack だけでも、似たような動作をさせることは可能なのですが、個人的には webpack はコンパイラとして使い、タスク管理は gulp などを使うというのがしっくりきます。
- npm
- 6.9.0
- gulp
- 4.0.2
- webpack
- 4.39.3
1. gulp、webpack をインストール
1-1. package.json の初期化
$ cd /path/to/gulp-webpack
$ npm init -y
処理が完了すると package.json が作られます。それをエディタで開き、description: "",の下部にある"main": "index.js",を削除し、そこに"private": true,を追記。
{
"name": "gulp-webpack",
"version": "1.0.0",
"description": "",
"main": "index.js", ← これを削除
"private": true, ← これを追加
~~~ 省略 ~~~
}
1-2. インストール
下記コマンドで、gulp、webpack をインストール。
webpack-stream は gulp で webpack を実行するために必要なプラグインです。
$ npm i gulp webpack webpack-cli webpack-stream --save-dev
2. エントリポイントとなるJSファイルを作成
下記構成でJSファイルを3つ作成。
/gulp-webpack
|- /node_modules
|- /src
|- /sub
|- test-1.js
|- test-2.js
|- test-3.js
|- package-lock.json
|- package.json
// sub/test-1.js
alert("Test 1");
// test-2.js
alert("Test 2");
// test-3.js
alert("Test 3");
3. webpack.config.js を作成
下記内容で webpack.config.js を作成。
const glob = require("glob");
// srcフォルダ下にある .js のファイルを抽出、オブジェクトに追加
const entries = {};
const srcFiles = `./src/**/*.js`;
glob.sync(srcFiles).map((file) => {
const key = file.replace("src/", "");
entries[key] = file;
});
module.exports = {
mode: "production",
entry: entries,
output: {
filename: "[name]"
}
};
4. gulpfile.js を作成
下記の内容で gulpfile.js を作成
const gulp = require("gulp");
const path = require("path");
const webpack = require("webpack");
const webpackStream = require("webpack-stream");
// webpack を実行するタスク
gulp.task("build-js", () => {
// webpack.config.js 読込
const webpackConfigPath = path.resolve(__dirname, "webpack.config.js");
delete require.cache[webpackConfigPath];
const webpackConfig = require(webpackConfigPath);
// webpack 実行
return webpackStream(webpackConfig, webpack).on("error", function(e) {
this.emit("end");
})
.pipe(gulp.dest("./dist"));
});
// src ディレクトリ内のjsファイルが更新されたら、上記 webpack のタスク実行
gulp.task("default", () => {
gulp.watch("./src/**/*.js", gulp.task("build-js"));
});
webpackを実行するタスクの箇所は下記サイトを参考にしました。
- webpack-streamでエラー発生時に処理を終了させずに監視を続ける方法(plumber不要) | WEMO
- https://wemo.tech/1659
webpack.config.js の読み込みを、タスクの関数内で行っているのは、毎回 webpack を行う直前に src ディレクトリを操作することで、監視中に追加したJSファイルも正常にコンパイルするためです。
delete require.cache[ ~ ] をしているのは、require() には読込内容をキャッシュする機能があり、delete しないと、2回目以降は再読込せずに、1回目の結果を返してしまうためです。
5. 動作確認
下記コマンドで、JSファイルの監視を開始します。
$ npx gulp
[21:23:45] Using gulpfile /path/to/gulp-webpack/gulpfile.js
[21:23:45] Starting 'default'...
上記のように Starting 'default' と表示されたら、 src 内にあるJSファイルのどれか1つを開き、変更を加えずにそのまま上書き保存してください。すると下記のように webpack が実行されます。
[21:23:55] Starting 'build-js'...
[21:23:56] Version: webpack 4.39.3
Built at: 2019-08-28 9:23:56 PM
Asset Size Chunks Chunk Names
./sub/test-1.js 945 bytes 0 [emitted] ./sub/test-1.js
./test-2.js 946 bytes 1 [emitted] ./test-2.js
./test-3.js 947 bytes 2 [emitted] ./test-3.js
Entrypoint ./sub/test-1.js = ./sub/test-1.js
Entrypoint ./test-2.js = ./test-2.js
Entrypoint ./test-3.js = ./test-3.js
[21:23:56] Finished 'build-js' after 385 ms
dist ディレクトリにコンパイルされたJSファイルが作成されていれば完了です。