Browserify を gulp の src() で使用する方法(エラー処理付)
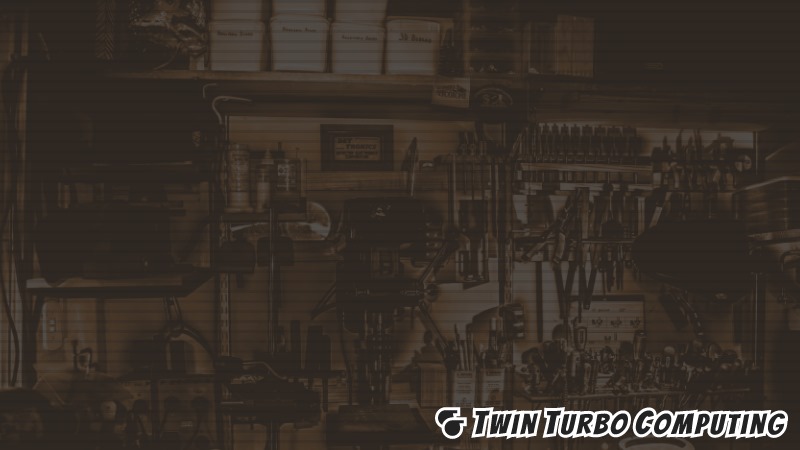
はじめに
今日は gulp の src() を使った Browserify 実行方法と、併せて Babel と terser を使う方法をご紹介します。
もし一部ファイルにだけ Browserify したい場合は、前回の「Browserify + gulp で一部 JS を除外して実行する方法」でご紹介していますので、併せてご覧ください。
- Node.js
- 14.17.0
- Browserify
- 17.0.0
- gulp
- 4.0.2
- plugin-error
- 1.0.1
- through2
- 4.0.2
1. 下準備
今回使用したファイルの構成と内容は以下の通りです。
gulpfile.js については「2. gulpfile.js 実装方法」でご紹介します。
/path/to/my-project/
├ public/
│ └ index.html
│
├ src/
│ ├ entries/
│ │ └ app.js
│ │
│ └ modules/
│ └ sample.js
│
├ gulpfile.js
└ package.json
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>Browserify Sample</title>
<script src="app.js"></script>
</head>
<body>
結果は console.log で出力
</body>
</html>
const { debug } = require('../modules/sample.js');
debug(`Hello, world!`);
module.exports = {
debug: (value) => {
console.log('Debug: ' + value);
}
}
{
"private": true,
"devDependencies": {
"browserify": "^17.0.0",
"gulp": "^4.0.2",
"plugin-error": "^1.0.1",
"through2": "^4.0.2"
}
}
あとは下記コマンドなどで上記 Node パッケージをインストールしてください。
cd /path/to/my-project/
npm i
2. gulpfile.js 実装方法
gulp での Browserify 実行は gulpfile.js を下記のようにすることで可能です。
下記サンプルでは src 内の .js ファイルを監視し、更新があった場合は Browserify しています。
const { dest, src, task, watch } = require('gulp');
const browserify = require('browserify');
const PluginError = require('plugin-error');
const through2 = require('through2');
// JS ソース
const jsSrc = `./src/entries/**/*.js`;
// JS 出力先
const jsDest = `./public`;
task('js-compile', () => {
return src(jsSrc)
// Browserify
.pipe(through2.obj((file, enc, callback) => {
browserify(file.path)
.bundle((err, buf) => {
// エラー処理
if (err !== null) {
return callback(new PluginError('browserify', err, {
showProperties: true,
}));
}
file.contents = buf;
callback(err, file);
});
}))
// public フォルダに出力
.pipe(dest(jsDest));
});
task('default', () => {
// src 内の JS を監視、更新時に js-compile を実行
watch('src/**/*.js', task('js-compile'));
});
- GitHub - rvagg/through2: Tiny wrapper around Node streams2 Transform to avoid explicit subclassing noise
- https://github.com/rvagg/through2#through2
- b.bundle(cb) - GitHub - browserify/browserify: browser-side require() the node.js way
- https://github.com/browserify/browserify#bbundlecb
- GitHub - gulpjs/plugin-error: Error handling for vinyl plugins. Just an abstraction of what's in gulp-util with minor reformatting.
- https://github.com/gulpjs/plugin-error#plugin-error
監視は下記コマンドで開始でき、監視終了は Ctrl + C です。
cd /path/to/my-project/
npx gulp
src 内の .js ファイルを上書き保存すると Browserify が実行され、public に app.js が生成されます。
3. Babel, terser を追加
Babel と terser は下記のようにすることで実行できます。
Babel は babelify を使用して、browserify の中で実行します。
cd /path/to/my-project/
npm i -D @babel/core @babel/preset-env babelify gulp-terser
const { dest, src, task, watch } = require('gulp');
const browserify = require('browserify');
const PluginError = require('plugin-error');
const through2 = require('through2');
// ↓ 追加
const terser = require('gulp-terser');
const jsSrc = `./src/entries/**/*.js`;
const jsDest = `./public`;
task('js-compile', () => {
return src(jsSrc)
.pipe(through2.obj((file, enc, callback) => {
browserify(file.path)
// ↓ 追加
.transform('babelify', { presets: ['@babel/preset-env'] })
.bundle((err, buf) => {
if (err !== null) {
return callback(new PluginError('browserify', err, {
showProperties: true,
}));
}
file.contents = buf;
callback(err, file);
});
}))
// ↓ 追加
.pipe(terser())
.pipe(dest(jsDest));
});
task('default', () => {
watch('src/**/*.js', task('js-compile'));
});
- GitHub - babel/babelify: Browserify transform for Babel
- https://github.com/babel/babelify#babelify-
- GitHub - terser/terser: JavaScript parser, mangler and compressor toolkit for ES6+
- https://github.com/terser/terser#readme
4. おわりに
gulp の src() を使って Browserify したいと思い、gulp-babel や gulp-terser のようなパッケージを探したのですが、メジャーと思われるものが見当たらなかったので、through2 で実装してみました。
gulpfile.js は資料が多くて、中身を知らなくても使えることが多いですが、pipe() や vinyl について調べると理解が深まると思います。
- Stream | Node.js v14.17.3 Documentation
- https://nodejs.org/dist/latest-v14.x/docs/api/stream.html#stream_readable_pipe_destination_options
- Vinyl | gulp.js
- https://gulpjs.com/docs/en/api/vinyl/